Engineering, Backend
Fixing Go’s Linker: An Unexpected Journey into ARM64, DWARF, and Linker Internals
16 February 2023 / Global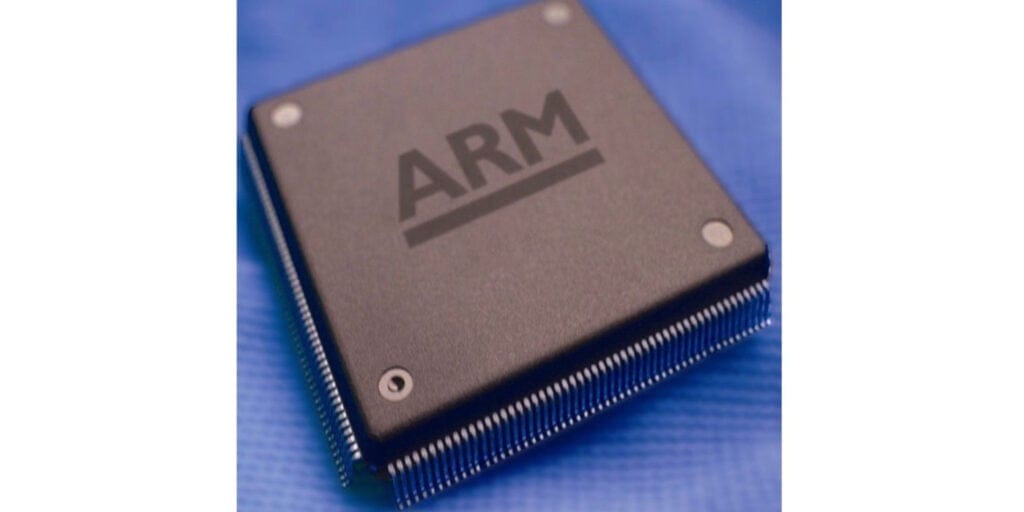
Introduction
We encountered an unusual problem recently at Uber with Golang™ debugging, as our engineers began transitioning to Apple® Silicon hardware, which uses the ARM64 Instruction Set Architecture (ISA), rather than the x86/AMD64 ISA many of us have been using for many years now. This required some rather complex debugging of the toolchain itself by Uber engineers. This post will showcase the analysis techniques, and dive into some topics including:
- DWARF
- ARM64 limitations
- The linking process and linker internals
- Low level inspection of object files and executables
Some engineers with Apple® M1s were reporting they were unable to set breakpoints or step through their Go